Maybe someone can pick up the errors in this? This is the raw code. It’s late and the pic from the scene.
Full Image : i61.tinypic.com/2i7rci9.jpg
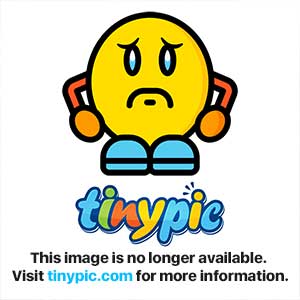
[code]// code to handle actual commans
void ExistenceClient::GenerateScene(const time_t ×eed, planet_rule planetrule)
{
/// play with rules
srand(timeseed); // use time as the rule
/// Define Resouces
ResourceCache* cache = GetSubsystem<ResourceCache>();
Renderer* renderer = GetSubsystem<Renderer>();
Graphics* graphics = GetSubsystem<Graphics>();
UI* ui = GetSubsystem<UI>();
FileSystem * filesystem = GetSubsystem<FileSystem>();
/// create variables (urho3d)
String InputDataFile;
/// Create Scene components
scene_-> CreateComponent<Octree>();
scene_-> CreateComponent<PhysicsWorld>();
scene_-> CreateComponent<DebugRenderer>();
/// Create skybox. The Skybox component is used like StaticModel, but it will be always located at the camera, giving the
/// illusion of the box planes being far away. Use just the ordinary Box model and a suitable material, whose shader will
/// generate the necessary 3D texture coordinates for cube mapping
Node* skyNode = scene_->CreateChild("Sky");
skyNode->SetScale(500.0f); // The scale actually does not matter
Skybox* skybox = skyNode->CreateComponent<Skybox>();
skybox->SetModel(cache->GetResource<Model>("Models/Box.mdl"));
skybox->SetMaterial(cache->GetResource<Material>("Materials/Skybox.xml"));
/// Create a Zone component for ambient lighting & fog control
Node* zoneNode = scene_->CreateChild("Zone");
Zone* zone = zoneNode->CreateComponent<Zone>();
Vector3 boundingBoxMin(-1000.0f,0,-1000.0f);
Vector3 boundingBoxMax(1000.0f,2000.0f,1000.0f);
/// change bounding box to something more accurate
zone->SetBoundingBox(BoundingBox(boundingBoxMin,boundingBoxMax));
zone->SetAmbientColor(Color(0.01f, 0.01f, .01f));
zone->SetFogColor(Color(1.0f, 1.0f, 1.0f));
zone->SetFogStart(0.0f);
zone->SetFogEnd(1000.0f);
zone->SetHeightFog (false);
/// Create a directional light to the world. Enable cascaded shadows on it
Node* lightNode = scene_->CreateChild("DirectionalLight");
lightNode->SetDirection(Vector3(0.6f, -1.0f, 0.8f));
Light* light = lightNode->CreateComponent<Light>();
light->SetLightType(LIGHT_DIRECTIONAL);
light->SetCastShadows(false);
light->SetShadowBias(BiasParameters(0.00025f, 0.5f));
light->SetShadowCascade(CascadeParameters(10.0f, 50.0f, 200.0f, 0.0f, 0.8f));
light->SetSpecularIntensity(0.0f);
light->SetBrightness(.9);
light->SetColor(Color(0.251f, 0.612f, 1.0f));
/// Create a directional light to the world. Enable cascaded shadows on it
Node* lightNode2 = scene_->CreateChild("DirectionalLight2");
Light* light2 = lightNode2->CreateComponent<Light>();
light2->SetLightType(LIGHT_DIRECTIONAL);
light2->SetCastShadows(true);
light2->SetShadowBias(BiasParameters(0.00025f, 0.5f));
light2->SetShadowCascade(CascadeParameters(10.0f, 50.0f, 200.0f, 0.0f, 0.8f));
light2->SetSpecularIntensity(2.0f);
light2->SetBrightness(.7);
light2->SetColor(Color(1.0f, 1.0f,.95f));
lightNode2->SetRotation(Quaternion(55.7392,0,0));
/// Generate Terrain
Node* terrainNode = scene_->CreateChild("Terrain");
Terrain* terrain = terrainNode->CreateComponent<Terrain>();
terrain->SetPatchSize(64);
terrain->SetSpacing(Vector3(2.0f, 0.8f, 2.0f)); // Spacing between vertices and vertical resolution of the height map
terrain->SetSmoothing(true);
terrain->SetCastShadows(true);
/// generatescene
terrain->GenerateProceduralHeightMap(planetrule);
terrain->SetMaterial(cache->GetResource<Material>("Materials/TerrainTriPlanar.xml"));
RigidBody* terrainbody = terrainNode->CreateComponent<RigidBody>();
CollisionShape* terrainshape = terrainNode->CreateComponent<CollisionShape>();
terrainbody->SetCollisionLayer(1);
terrainshape->SetTerrain();
/// Create a scene node for the camera, which we will move around
/// The camera will use default settings (1000 far clip distance, 45 degrees FOV, set aspect ratio automatically)
cameraNode_ = new Node(context_);
cameraNode_ = scene_->CreateChild("Camera");
cameraNode_->CreateComponent<Camera>();
Camera* camera = cameraNode_->CreateComponent<Camera>();
camera->SetFarClip(1000.0f);
// Set an initial position for the camera scene node above the ground
cameraNode_->SetPosition(Vector3(0.0f, 0.0f, 0.0f));
SharedPtr<Viewport> viewport(new Viewport(context_, scene_, cameraNode_->GetComponent<Camera>()));
renderer->SetViewport(0, viewport);
Vector3 position(0.0f,0.0f);
position.y_ = terrain->GetHeight(position) + 1.0f;
/// Position character
Node * characternode_ = scene_->CreateChild("Character");
characternode_->SetPosition(Vector3(0.0f, position.y_ , 0.0f));
/// Create chacter
CreateCharacter();
/// Load main UI area
loadSceneUI();
/// rest of UI
loadHUDFile("Resources/UI/MainTopBarWindow.xml",0,0);
loadHUDFile("Resources/UI/PlayerInfoWindow.xml",0,34);
/// Add a object to the seen - Pod Node
Node * PodNode1 = scene_ -> CreateChild("podNode");
StaticModel* PodObject1 = PodNode1 ->CreateComponent<StaticModel>();
PodObject1->SetModel(cache->GetResource<Model>("Resources/Models/pod.mdl"));
PodObject1->ApplyMaterialList("Resources/Models/pod.txt");
PodObject1->SetCastShadows(true);
Vector3 position2(4.0f,4.0f);
position2.y_ = terrain->GetHeight(position2);
PodNode1->SetPosition(Vector3(4.0f, position2.y_+.6f , 4.0f));
RigidBody* PodBody= PodNode1->CreateComponent<RigidBody>();
CollisionShape* Podshape = PodNode1->CreateComponent<CollisionShape>();
Podshape->SetTriangleMesh (cache->GetResource<Model>("Resources/Models/pod.mdl"));
PodBody->SetCollisionLayer(1);
Podshape ->SetLodLevel(1);
return;
}
// load a HUD File and sets it’s position
bool ExistenceClient::loadHUDFile(const char * filename, const int positionx, const int positiony)
{
/// Get resources
ResourceCache * cache = GetSubsystem();
FileSystem * filesystem = GetSubsystem();
UI* ui_ = GetSubsystem();
/// get current root
UIElement * RootUIElement = ui_->GetRoot();
UIElement * HUDFileElement= new UIElement(context_);
UIElement * playerInfoHudElement= new UIElement(context_);
/// Configure resources
XMLElement hudElement;
/// Configure string to Urho friendly
String filenameHUD = String(filename);
/// Load Resource
XMLFile* hudFile= cache->GetResource<XMLFile>(filenameHUD);
/// Get root element XML
hudElement = hudFile->GetRoot();
/// Add a min top bar
HUDFileElement-> LoadXML(hudElement);
/// Add a uielement for the bar
RootUIElement -> AddChild(HUDFileElement);
/// Position the window
HUDFileElement -> SetPosition(positionx,positiony);
HUDFileElement->SetStyleAuto();
return true;
}
void ExistenceClient::loadSceneUI(void)
{
// get resources
ResourceCache* cache = GetSubsystem<ResourceCache>();
Renderer* renderer = GetSubsystem<Renderer>();
Graphics* graphics = GetSubsystem<Graphics>();
UI* ui = GetSubsystem<UI>();
FileSystem * filesystem = GetSubsystem<FileSystem>();
ui->Clear();
/// Get rendering window size as floats
float width = (float)graphics->GetWidth();
float height = (float)graphics->GetHeight();
UIElement * menuUIElements = new UIElement (context_);
UIElement * quickmenu = new UIElement (context_);
// buttons and menus needed
Button * logoutsceneButton = new Button(context_);
Text * logoutsceneText = new Text(context_);
// Login screen - Create the Window and add it to the UI's root node
window_= new Window(context_);
// position menu
quickmenu->SetPosition(width-158,height-100);
quickmenu->SetLayout(LM_FREE, 6, IntRect(0, 0, 158, 32));
quickmenu->SetAlignment(HA_LEFT, VA_TOP);
quickmenu->SetMinSize(158, 32);
quickmenu->SetPriority(1);
// Set Window size and layout settings
window_->SetPosition(0,0);
window_->SetMinSize(158, 32);
window_->SetLayout(LM_FREE, 6, IntRect(0, 0, 158, 32));
window_->SetAlignment(HA_LEFT, VA_TOP);
window_->SetMovable(false);
logoutsceneButton -> SetLayout(LM_FREE, 6, IntRect(0, 0, 158,32));
logoutsceneButton -> SetAlignment(HA_LEFT, VA_TOP);
logoutsceneButton -> SetFixedSize(158, 32);
logoutsceneButton -> SetPosition(0, 0);
logoutsceneButton -> SetName("logoutsceneButton");
logoutsceneButton -> SetOpacity(.8);
logoutsceneText -> SetPosition(64, 0);
logoutsceneText -> SetAlignment(HA_LEFT, VA_CENTER);
logoutsceneText -> SetTextAlignment(HA_LEFT);
logoutsceneText -> SetText("LOGOUT");
/// Set font and text color
logoutsceneText -> SetFont(cache->GetResource<Font>("Fonts/Anonymous Pro.ttf"), 14);
menuUIElements -> SetPosition(0,0);
menuUIElements -> SetMinSize(158,32);
menuUIElements -> SetAlignment(HA_LEFT, VA_TOP);
menuUIElements -> SetOpacity(.8);
/// Each Menu element have a button and text
menuUIElements -> AddChild(logoutsceneButton);
menuUIElements -> AddChild(logoutsceneText);
/// Add menu elements
window_->AddChild(menuUIElements);
/// Add menu to UIElement
quickmenu->AddChild(window_);
/// Add to root
uiRoot_->AddChild(quickmenu);
menuUIElements->SetStyleAuto();
quickmenu->SetStyleAuto();
window_->SetStyleAuto();
logoutsceneButton -> SetStyle("logoutsceneButton");
return;
}
[/code]